Building distributed applications is a crucial aspect of modern software development. As the demand for scalable and fault-tolerant systems continues to grow, developers are turning to frameworks like Gin to create robust distributed applications. In this article, we will explore seven ways to build distributed applications in Gin, highlighting the benefits and challenges of each approach.
Distributed applications are designed to run on multiple machines, communicating with each other to achieve a common goal. This architecture provides numerous benefits, including increased scalability, improved fault tolerance, and enhanced performance. However, building distributed applications can be complex, requiring careful consideration of communication protocols, data consistency, and system reliability.
Gin is a popular Go framework that provides a lightweight and flexible way to build web applications. Its modular design and extensive library make it an ideal choice for building distributed applications.
1. Microservices Architecture
One of the most popular ways to build distributed applications is using a microservices architecture. This approach involves breaking down a monolithic application into smaller, independent services that communicate with each other using APIs.
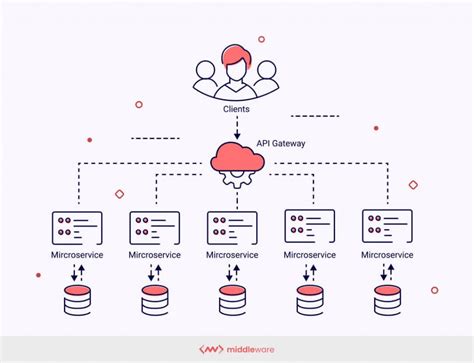
In Gin, you can create microservices by defining separate Go packages for each service. Each package can have its own main function, allowing you to run each service independently.
// user-service/main.go
package main
import (
"github.com/gin-gonic/gin"
"net/http"
)
func main() {
r := gin.Default()
r.GET("/users", getUser)
http.ListenAndServe(":8080", r)
}
func getUser(c *gin.Context) {
// Get user logic
}
2. Service-Oriented Architecture (SOA)
Service-Oriented Architecture (SOA) is another approach to building distributed applications. In SOA, applications are designed as a collection of services that communicate with each other to achieve a common goal.

In Gin, you can create SOA-based applications by defining services as separate Go packages. Each package can have its own interface, allowing you to define a contract for the service.
// user-service/user.go
package user
type UserService interface {
GetUser(id string) (*User, error)
}
type User struct {
ID string
Name string
}
func (u *UserService) GetUser(id string) (*User, error) {
// Get user logic
}
3. Event-Driven Architecture (EDA)
Event-Driven Architecture (EDA) is a design pattern that involves producing and consuming events to communicate between services.

In Gin, you can create EDA-based applications by using a message broker like RabbitMQ or Apache Kafka. Each service can produce and consume events using the message broker.
// user-service/main.go
package main
import (
"github.com/gin-gonic/gin"
"github.com/streadway/amqp"
)
func main() {
// Connect to RabbitMQ
conn, err := amqp.Dial("amqp://guest:guest@localhost:5672/")
if err!= nil {
panic(err)
}
defer conn.Close()
// Create a channel
ch, err := conn.Channel()
if err!= nil {
panic(err)
}
defer ch.Close()
// Create a queue
q, err := ch.QueueDeclare(
"user_queue",
true,
false,
false,
false,
nil,
)
if err!= nil {
panic(err)
}
// Consume events from the queue
msgs, err := ch.Consume(
q.Name,
"",
true,
false,
false,
false,
nil,
)
if err!= nil {
panic(err)
}
go func() {
for d := range msgs {
// Process event
}
}()
// Start the Gin server
r := gin.Default()
http.ListenAndServe(":8080", r)
}
4. RESTful API
RESTful API is a popular way to build distributed applications. In RESTful API, resources are exposed as URIs, and clients can interact with these resources using HTTP methods.

In Gin, you can create RESTful APIs by defining routes for each resource.
// user-service/main.go
package main
import (
"github.com/gin-gonic/gin"
"net/http"
)
func main() {
r := gin.Default()
r.GET("/users", getUser)
r.POST("/users", createUser)
http.ListenAndServe(":8080", r)
}
func getUser(c *gin.Context) {
// Get user logic
}
func createUser(c *gin.Context) {
// Create user logic
}
5. GraphQL
GraphQL is a query language for APIs. It allows clients to specify exactly what data they need, reducing the amount of data transferred over the network.

In Gin, you can create GraphQL APIs using the github.com/graphql-go/graphql
package.
// user-service/main.go
package main
import (
"github.com/graphql-go/graphql"
"github.com/graphql-go/graphql/gqltesting"
)
func main() {
// Define the GraphQL schema
fields := graphql.Fields{
"users": &graphql.Field{
Type: graphql.NewList(graphql.String),
Resolve: func(p graphql.ResolveParams) (interface{}, error) {
// Get users logic
},
},
}
rootQuery := graphql.ObjectConfig{
Name: "Query",
Fields: fields,
}
schema, err := graphql.NewSchema(graphql.SchemaConfig{
Query: graphql.NewObject(rootQuery),
})
if err!= nil {
panic(err)
}
// Start the Gin server
r := gin.Default()
http.ListenAndServe(":8080", r)
}
6. gRPC
gRPC is a high-performance RPC framework that allows you to build distributed applications.

In Gin, you can create gRPC services using the google.golang.org/grpc
package.
// user-service/main.go
package main
import (
"context"
"fmt"
"net"
"google.golang.org/grpc"
pb "user-service/proto"
)
func main() {
// Create a gRPC server
s := grpc.NewServer()
pb.RegisterUserServiceServer(s, &userService{})
// Start the server
lis, err := net.Listen("tcp", ":8080")
if err!= nil {
panic(err)
}
s.Serve(lis)
}
type userService struct{}
func (s *userService) GetUser(ctx context.Context, req *pb.GetUserRequest) (*pb.GetUserResponse, error) {
// Get user logic
}
7. WebSockets
WebSockets is a bi-directional communication protocol that allows you to build real-time applications.

In Gin, you can create WebSocket applications using the github.com/gorilla/websocket
package.
// user-service/main.go
package main
import (
"fmt"
"log"
"net/http"
"github.com/gin-gonic/gin"
"github.com/gorilla/websocket"
)
func main() {
// Create a WebSocket server
var upgrader = websocket.Upgrader{} // use default options
r := gin.Default()
r.GET("/ws", func(c *gin.Context) {
c.Header("Access-Control-Allow-Origin", "*")
c.Header("Access-Control-Allow-Methods", "GET,POST,OPTIONS")
c.Header("Access-Control-Allow-Headers", "Content-Type,Content-Length,Accept-Encoding,X-CSRF-Token,Authorization,accept,origin,Cache-Control,X-Requested-With")
// Upgrade the connection to a WebSocket connection
ws, err := upgrader.Upgrade(c.Writer, c.Request, nil)
if err!= nil {
log.Println(err)
return
}
defer ws.Close()
// Handle WebSocket messages
for {
messageType, r, err := ws.NextReader()
if err!= nil {
log.Println(err)
return
}
ws.WriteMessage(messageType, r)
}
})
http.ListenAndServe(":8080", r)
}
In this article, we explored seven ways to build distributed applications in Gin. Each approach has its benefits and challenges, and the choice of approach depends on the specific requirements of your application. By understanding these approaches, you can design and build scalable and fault-tolerant distributed applications using Gin.
What approach do you think is best for building distributed applications in Gin? Share your thoughts in the comments below!








What is a distributed application?
+A distributed application is a software application that runs on multiple computers or machines, communicating with each other to achieve a common goal.
What are the benefits of building distributed applications?
+The benefits of building distributed applications include increased scalability, improved fault tolerance, and enhanced performance.
What is Gin?
+Gin is a popular Go framework that provides a lightweight and flexible way to build web applications.